To implement a 360-degree direction listener for mouse inputs in Python, you can use the pynput
library along with some trigonometry to calculate the direction. Here’s a step-by-step guide on how to achieve this:
- Install the
pynput
library if you haven’t already:
pip install pynput
- Create a Python script with the following code to listen to mouse inputs and print the direction:
from pynput.mouse import Listener
import math
# Global variables to store the previous mouse position
prev_x = None
prev_y = None
def on_move(x, y):
global prev_x, prev_y
if prev_x is not None and prev_y is not None:
dx = x - prev_x
dy = y - prev_y
# Calculate the angle in radians
angle = math.atan2(dy, dx)
# Convert radians to degrees
angle_degrees = math.degrees(angle)
# Normalize angle to be between 0 and 360 degrees
if angle_degrees < 0:
angle_degrees += 360
print(f"Mouse moved in direction: {angle_degrees:.2f} degrees")
prev_x = x
prev_y = y
def on_click(x, y, button, pressed):
pass
def on_scroll(x, y, dx, dy):
pass
# Create a listener for mouse events
with Listener(on_move=on_move, on_click=on_click, on_scroll=on_scroll) as listener:
listener.join()
This code listens to mouse movements, calculates the direction of movement in degrees, and prints it to the terminal. The atan2
function from the math
module is used to calculate the angle between the current and previous mouse positions.
You can further extend this code to save the data or plot it using a data visualization library like matplotlib
for your long-term goals.
Example Output:
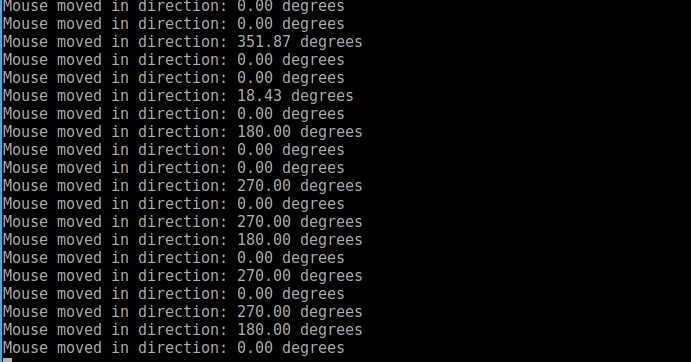